先看效果,就是左右滑屏的效果
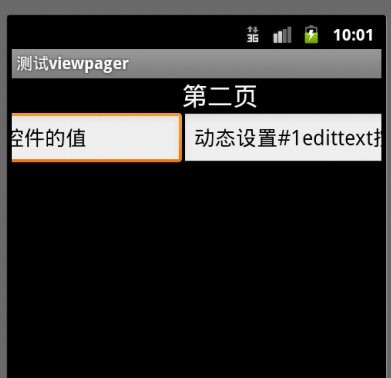
具体实现详解
android compatibility package, revision 3在7月份发布后,其中有个ViewPager引起了我的注意
官方的描述:
请参考:http://developer.android.com/sdk/compatibility-library.html#Notes
ViewPager的下载与安装
首先通过SDK Manager更新最新版android compatibility package, revision 3
更新后,在eclipse中工程上点击右键,选择android tools -> add compatibility library即可完成安装
实际上就是一个jar包,手工导到工程中也可
jar包所在位置是\android-sdk\extras\android\compatibility\v4\android-support-v4.jar
至此准备环境已经ok
下边还是通过代码进行说话吧
准备布局文件
viewpager_layout.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent" android:orientation="vertical">
<!-- 此处需要给出全路径 -->
<android.support.v4.view.ViewPager
android:id="@+id/viewpagerLayout" android:layout_height="fill_parent" android:layout_width="fill_parent"/>
</LinearLayout>
layout1.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent" android:orientation="vertical">
<TextView android:textAppearance="?android:attr/textAppearanceLarge" android:layout_height="wrap_content" android:id="@+id/textView1" android:layout_width="fill_parent" android:text="第一页"></TextView>
<EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/editText1">
<requestFocus></requestFocus>
</EditText>
</LinearLayout>
layout2.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent" android:orientation="vertical">
<TextView android:textAppearance="?android:attr/textAppearanceLarge" android:layout_height="wrap_content" android:id="@+id/textView1" android:layout_width="fill_parent" android:text="第二页"></TextView>
<EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/editText1">
<requestFocus></requestFocus>
</EditText>
</LinearLayout>
layout3.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent" android:orientation="vertical">
<TextView android:textAppearance="?android:attr/textAppearanceLarge" android:layout_height="wrap_content" android:id="@+id/textView1" android:layout_width="fill_parent" android:text="第三页"></TextView>
<EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/editText1">
<requestFocus></requestFocus>
</EditText>
</LinearLayout>
主程序
package a.b;
import java.util.ArrayList;
import java.util.List;
import android.app.Activity;
import android.os.Bundle;
import android.os.Parcelable;
import android.support.v4.view.PagerAdapter;
import android.support.v4.view.ViewPager;
import android.support.v4.view.ViewPager.OnPageChangeListener;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.EditText;
public class TestViewPager extends Activity {
private ViewPager myViewPager;
private MyPagerAdapter myAdapter;
private LayoutInflater mInflater;
private List<View> mListViews;
private View layout1 = null;
private View layout2 = null;
private View layout3 = null;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.viewpager_layout);
myAdapter = new MyPagerAdapter();
myViewPager = (ViewPager) findViewById(R.id.viewpagerLayout);
myViewPager.setAdapter(myAdapter);
mListViews = new ArrayList<View>();
mInflater = getLayoutInflater();
layout1 = mInflater.inflate(R.layout.layout1, null);
layout2 = mInflater.inflate(R.layout.layout2, null);
layout3 = mInflater.inflate(R.layout.layout3, null);
mListViews.add(layout1);
mListViews.add(layout2);
mListViews.add(layout3);
//初始化当前显示的view
myViewPager.setCurrentItem(1);
//初始化第二个view的信息
EditText v2EditText = (EditText)layout2.findViewById(R.id.editText1);
v2EditText.setText("动态设置第二个view的值");
myViewPager.setOnPageChangeListener(new OnPageChangeListener() {
@Override
public void onPageSelected(int arg0) {
Log.d("k", "onPageSelected - " + arg0);
//activity从1到2滑动,2被加载后掉用此方法
View v = mListViews.get(arg0);
EditText editText = (EditText)v.findViewById(R.id.editText1);
editText.setText("动态设置#"+arg0+"edittext控件的值");
}
@Override
public void onPageScrolled(int arg0, float arg1, int arg2) {
Log.d("k", "onPageScrolled - " + arg0);
//从1到2滑动,在1滑动前调用
}
@Override
public void onPageScrollStateChanged(int arg0) {
Log.d("k", "onPageScrollStateChanged - " + arg0);
//状态有三个0空闲,1是增在滑行中,2目标加载完毕
/**
* Indicates that the pager is in an idle, settled state. The current page
* is fully in view and no animation is in progress.
*/
//public static final int SCROLL_STATE_IDLE = 0;
/**
* Indicates that the pager is currently being dragged by the user.
*/
//public static final int SCROLL_STATE_DRAGGING = 1;
/**
* Indicates that the pager is in the process of settling to a final position.
*/
//public static final int SCROLL_STATE_SETTLING = 2;
}
});
}
private class MyPagerAdapter extends PagerAdapter{
@Override
public void destroyItem(View arg0, int arg1, Object arg2) {
Log.d("k", "destroyItem");
((ViewPager) arg0).removeView(mListViews.get(arg1));
}
@Override
public void finishUpdate(View arg0) {
Log.d("k", "finishUpdate");
}
@Override
public int getCount() {
Log.d("k", "getCount");
return mListViews.size();
}
@Override
public Object instantiateItem(View arg0, int arg1) {
Log.d("k", "instantiateItem");
((ViewPager) arg0).addView(mListViews.get(arg1),0);
return mListViews.get(arg1);
}
@Override
public boolean isViewFromObject(View arg0, Object arg1) {
Log.d("k", "isViewFromObject");
return arg0==(arg1);
}
@Override
public void restoreState(Parcelable arg0, ClassLoader arg1) {
Log.d("k", "restoreState");
}
@Override
public Parcelable saveState() {
Log.d("k", "saveState");
return null;
}
@Override
public void startUpdate(View arg0) {
Log.d("k", "startUpdate");
}
}
}
在实机上测试后,非常流畅,这也就是说官方版的左右滑屏控件已经实现
目前,关于viewpager的文章非常少,本文是通过阅读viewpager源代码分析出的写法
当然此文章仅是抛砖引玉,而且属于框架式程序,目的就是让读者了解API的基本用法
希望这篇原创文章对大家有帮助
欢迎感兴趣的朋友一起讨论
共同学习,共同进步
另外,ViewPager的注释上有这么一段话,大体意思是该控件目前属于早期实现,后续会有修改
02
|
*
Layout manager that allows the user to flip left and right
|
03
|
*
through pages of data. You supply an implementation of a
|
04
|
*
{@link PagerAdapter} to generate the pages that the view shows.
|
06
|
*
< p >Note
this class is currently under early design and
|
07
|
*
development. The API will likely change in later updates of
|
08
|
*
the compatibility library, requiring changes to the source code
|
09
|
*
of apps when they are compiled against the newer version.</ p >
|
分享到:
相关推荐
滑屏效果demo,支持左右滑屏,使用viewpager实现的
Android利用ViewPager实现类微信的左右滑动效果,详细代码注解解析setOnPageChangeListener各参数意义以及使用方法
点击缩略图查看大图,支持滑动切换图片,缩放图片,封装成了一个工具类,基本两行代码实现上面需求。 代码很清晰,可以自已微调。
Android ViewPager功能实例源码,实现手机左右滑屏切换效果,这种效果在Android手机中非常常见,界面UI设计必备的小技巧,本效果使用ViewPager实现,在安卓虚拟机上打开本源码后,可用鼠标来模拟拖动界面。
Android ViewPager左右滑动翻页。 适用于,第一次安装应用时的新手指导,及图片推荐浏览等。
类似PopupWindow的弹框DialogFragment实现TabLayout+ViewPager多个Fragment左右滑动切换
此程序是为了实现滑屏功能,可以用于欢迎界面,也可以是广告滑屏,初学viewpager可以使用学习一下哦!
用ViewPager实现手动切换,用Timer + Handler实现自动切换,左右完美无限滑动效果非常平滑,不卡,哈哈~Fragment里面我是写了文字,大家根据自己的需求放图片,设置点击事件等等。代码都有详细的注释,如果导入工程...
各种方向的ViewPager层叠卡片
android viewpager 实现了左右无限循环滑动 并且内附可自定义的小圆点指示器
使用ViewPager实现图片轮播效果,包括能自动轮播,左右无限制滑动,本人亲测,请放心下载
一款android viewpager 自定义滑动动画效果,很多种效果有你想要的,还可以理解之后自己写出自己想要的效果,很简单,调用就一段代码,不影响性能
ViewPagerCards 一分钟实现ViewPager卡片
Android ViewPager指示器,一行代码实现指示器效果
android左右滑动抽屉+ViewPager
http://blog.csdn.net/knight1996/article/details/79018054博客的参考代码
android viewpager实现微信tab简单切换
Android-Idea博客:Android中如何使用ViewPager实现类似laucher左右拖动效果源码
Android自定义View实现ViewPager指示器
安卓Android源码——ViewPager-实现左右两个屏幕的切换.zip